Today we will dive a little deeper and cover topics namely Conditional statements — If-Else, ELIF, Nested IF statements as well as Looping Statements including FOR and While Loops
Before we start: Python relies on indentation (whitespace at the beginning of a line) to define scope in the code. Other programming languages often use curly-brackets for this purpose. Improper indentations will lead to errors.
IF Statement
It is used to decide whether a certain statement or block of statements will be executed or not i.e. if a certain condition is true then a block of statement is executed otherwise not.
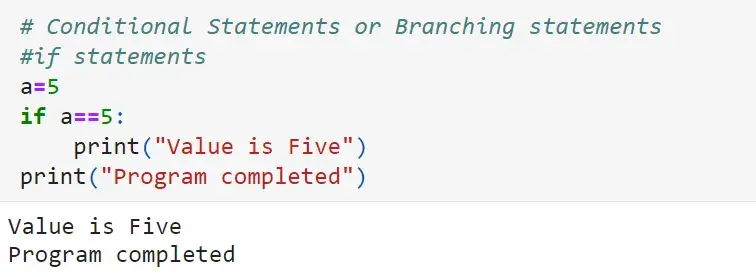
Short Hand IF Statement
If you have only one statement to execute, you can put it on the same line as the if statement.

IF - ELSE Statement
The if statement alone tells us that if a condition is true it will execute a block of statements and if the condition is false it won’t. We can use the else statement with if statement to execute a block of code when the condition is false.
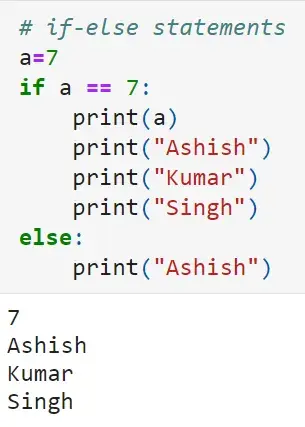
Short Hand IF - ELSE Statement
If you have only one statement to execute, one for if, and one for else, you can put it all on the same line.

IF - ELIF - ELSE Statement
The if statements are executed from the top down. As soon as one of the conditions controlling the if is true, the statement associated with that if is executed, and the rest of the ladder is bypassed. If none of the conditions is true, then the final else statement will be executed.
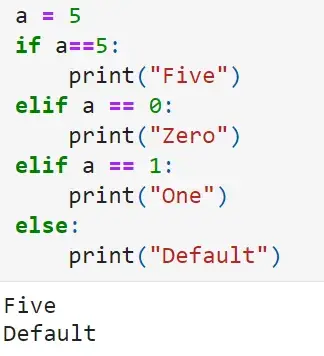
Joke of the Blog: Programming is like a “Choose your adventure game” except every path leads you to a Stack Overflow question from 2013 describing the same bug, with no answer
NESTED IF Statement
Nested if statements means an if statement inside another if statement.
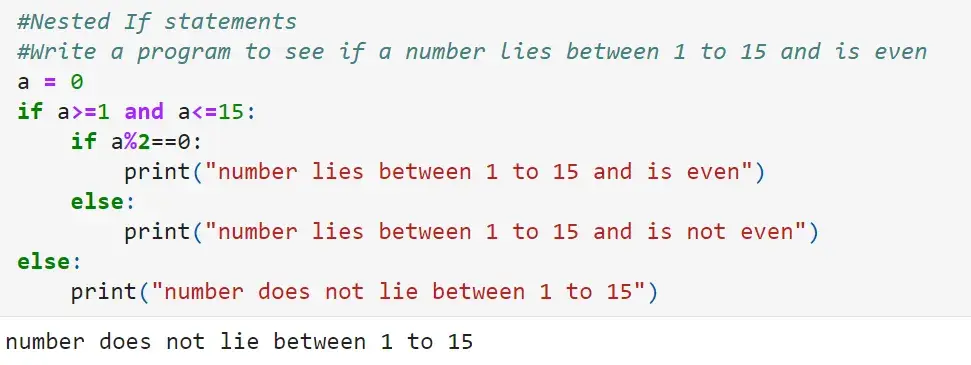
IF Statement with Logical Operators
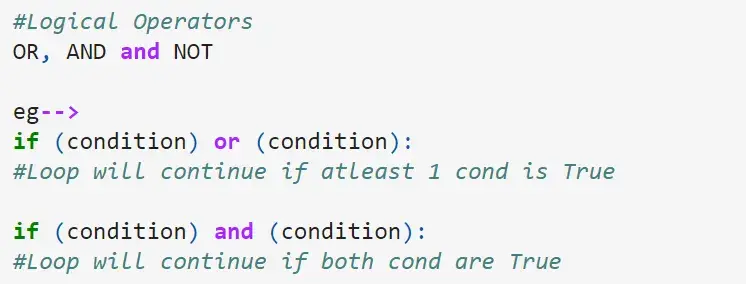
IF Statements with Pass Statement
IF statements cannot be empty, but if you for some reason have an IF statement with no content, put in the “pass” statement to avoid getting an error.
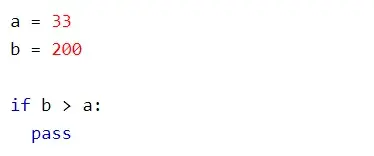
While Loop Statements
A while loop statement in Python programming language repeatedly executes a target statement as long as a given condition is true.
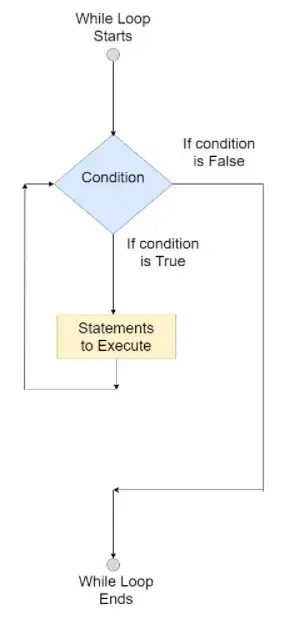

Let’s now try our hand at a real problem statement. We will write a program to check if a number is Armstrong or not.
A number is said to be Armstrong if the sum of the cube of the digits of the number is equal to the original number itself.
Consider 153 → cube(1)+cube(5)+cube(3) = 1+125+27 which is equal to 153.
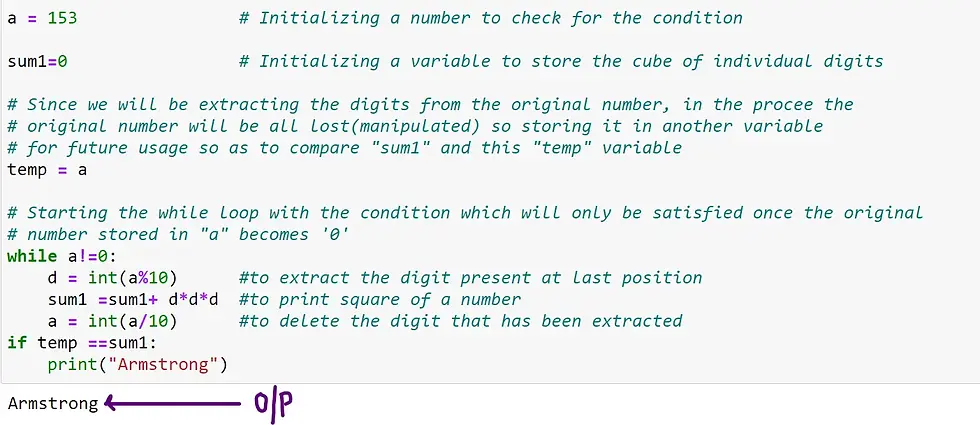
I will now break down the logic:
a = 153
sum1 = 0
temp = 153
Firstly the above 3 variables are initialized
Then the condition in while loop is executed and checked and if it is satisfied then only the loop will be executed
Condition for the loop to start: The loop will be iterated till the value of variable ‘a’ becomes ‘0’
In the loop, variable ‘d’ is used to extract the digits and is a
‘local’ variable that is it’s authority is valid only inside the
while loop and can only be used inside the loop and every time an
iteration of the loop is completed, it’s value will be reset again
Before First Iteration: a = 153, sum1 = 0 and temp = 153
Condition for while loop is checked and is satisfied as a is not equal to 0
# 1st Iteration:
d = 153%10 = 3
sum1 = 0+ 3*3*3 = 27
a = 153/10 = 15
After 1st Iteration:
a = 15, sum1 = 27 and temp = 153
Again the while loop’s condition is checked. Since a is still not equal to 0.
# 2nd Iteration:
d = 15%10 = 5
sum1 = 27+5*5*5 = 152
a = 15/10 = 1
After 2nd Iteration: a = 1, sum1 = 152 and temp = 153 Again the while loop’s condition is checked. Since a is still not equal to 0.
# 3rd Iteration:
d = 1%10 = 1
sum1 = 152+1*1*1 = 153
a = 1/10 = 0
After 3rd Iteration: a = 0, sum1 = 153 and temp = 153 Again the while loop’s condition is checked.
Since a is equal to 0, the loop ends and the next if condition is executed. This if statement compares the value of temp and sum1 and prints “Armstrong” when the condition is satisfied.
You can try your Hand at these for Practice: 1.) Write a Program to print prime numbers between 587 to 999 (both included) 2.) Write a Program to check if a number is palindrome or not. (A number is said to be palindrome if the reverse of the number is equal to the original number)
Eg → original numbers: 121,168
reverse : 121,861
“121” is Palindrome
“168” is not Palindrome
For Statement
The for loop is used to iterate over a sequence (list, tuple, string) or other iterable objects. This iteration over a given sequence is called traversal.

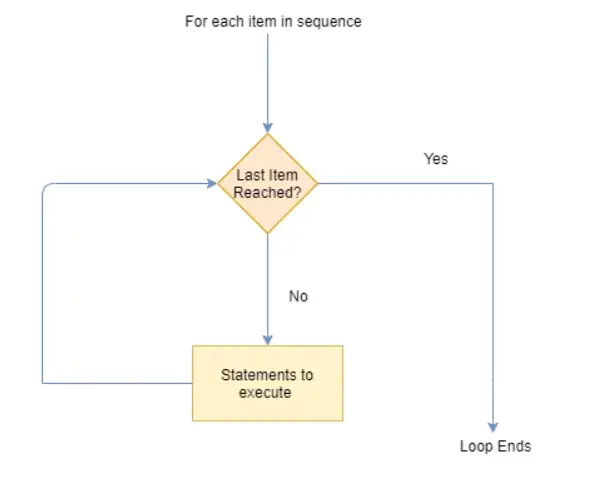

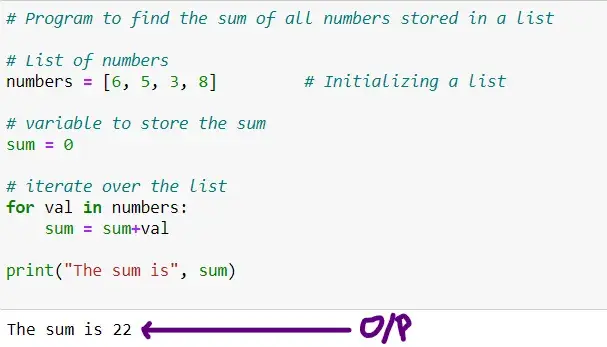
I will now break down the logic:
First we will create a ‘list’ data structure with some random numbers numbers = [6,5,3,8]
Initializing a variable as ‘sum’ to store the sum of numbers in the list sum = 0
Starting with the For loop Firstly a variable ‘val’ is declared which will be our iterator variable for the loop The loop will end once the whole list is traversed
First Iteration:
sum = 0+6 = 6
Second Iteration:
sum = 6+5 = 11
Third Iteration:
sum = 11+3 = 14
Fourth Iteration:
sum = 14+8 = 22
Loop Ends as there are no elements present in the list anymore
The sum is now printed as 22
You can try your Hand at these for Practice:
Python loops Practice Questions — CS-IP-Learning-Hub
Click on the above link to practice “For Loop”
If you are starting out today, don’t be afraid. It’s never too late. There’s a ton of material on the internet to learn for free and I am happy I was able to help you in the process. You can check out this link to read about IDE, data types, operators and variable declaration in python.
Comentarios