Agenda
What is IDE?
Data Types
Variables
Operators
What is IDE and where to code?
Before we begin with learning a programming language, we should be well ready with the prerequisites for the same.
An IDE (or Integrated Development Environment) is a program which is dedicated to software development. They are comprised of several tools specifically designed for software development.
To download an IDE, please click here. This link consists of a list of IDEs with their features mentioned as well as links to download them.
Which Python version we will be working on? Python 3.x. I will be using Jupyter Notebook to illustrate examples and explain the functionality. feel free to use any of the IDE
Data Types
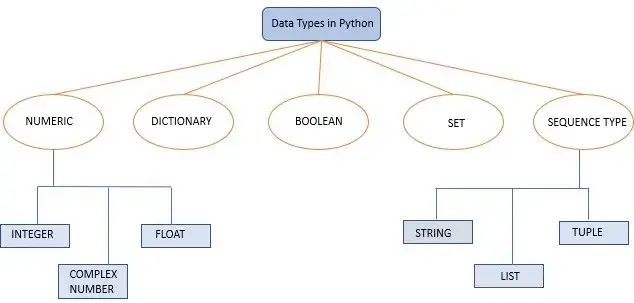
It refers to the classification of the data stored inside a data item. Primarily we’ll focus on the3 main types namely:
i) String → It is used to store character/sentences and can be created using single/double/triple quotes.
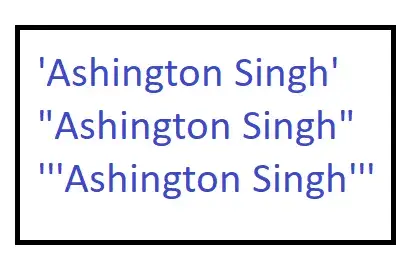
ii) Float → It is used to store numerical values with decimal.
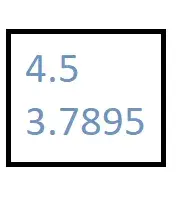
iii) Integer → It is used to store numerical values without the decimals
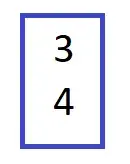
Note: To see data type of any object, we can use type() function
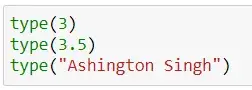
Variable Declaration
Python has no command for declaring a variable. A variable is created the moment you first assign a value to it.
1. Creating Variables
x = 5
y = “John”
2. Casting Variables → It is used to specify the data type of a variable
x = str(3) # x will be ‘3’
y = int(3) # y will be 3
z = float(3) # z will be 3.0
3. Variable names are case-sensitive. A variable can have a short name or a more descriptive name.
Rules for Python variables:
A variable name must start with a letter or the underscore character
A variable name cannot start with a number
A variable name can only contain alpha-numeric characters and underscores (A-z, 0–9, and _
Variable names are case-sensitive (age, Age and AGE are three different variables)
a = 4
A = “Sally”
#A will not overwrite a
4. Assign multiple values
# Python allows assigning values to multiple variables in one line
x, y, z = “Orange”, “Banana”, “Cherry”
5. Assign same value to multiple variables
x = y = z = “Orange”
6. Concept of Global Variables
These are the variables that are created outside of a function
x = “ashington”
def goof_up():
print(“Our savior is “ + x)
goof_up()
To create a global variable inside a function, you can use the ‘global’ keyword.
def goof_up():
global x
x = “awesome”
goof_up()
print(“Ashington is “ + x)
Operators
Arithmetic Operators
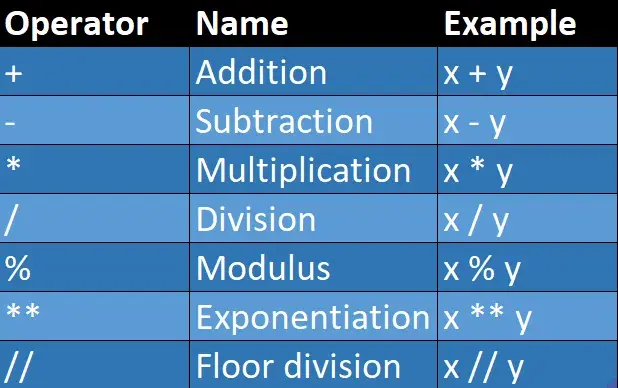
Modulus is used to get remainder of a division
Exponentiation is used to get power on x raised to y
Floor Division is used to divide 2 numbers and remove the decimal part
Assignment Operators
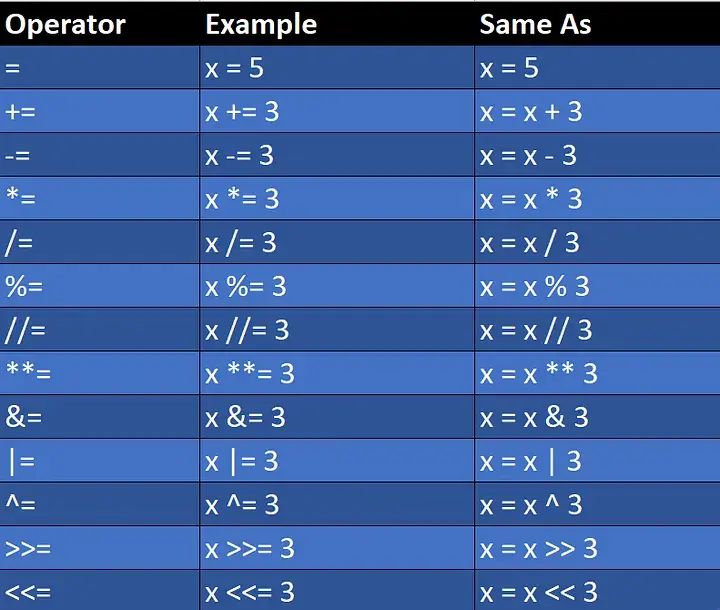
Comparison Operators
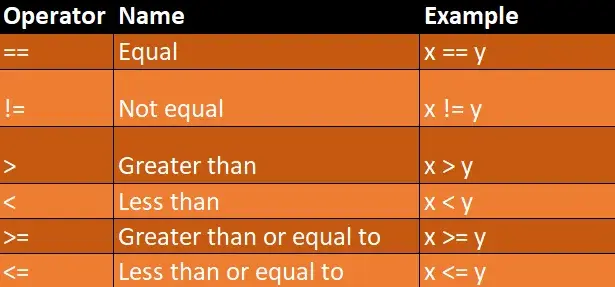
Logical Operators
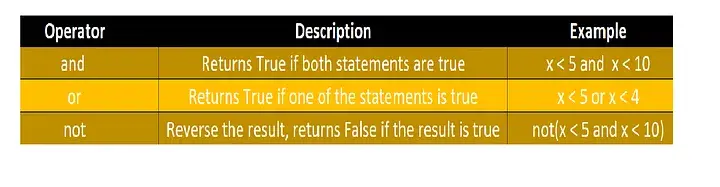
Membership Operators
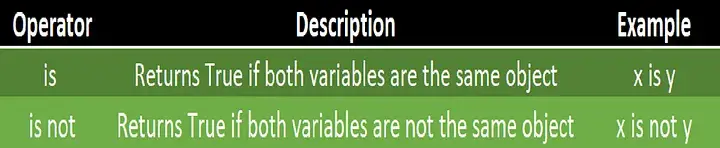
Bitwise Operators
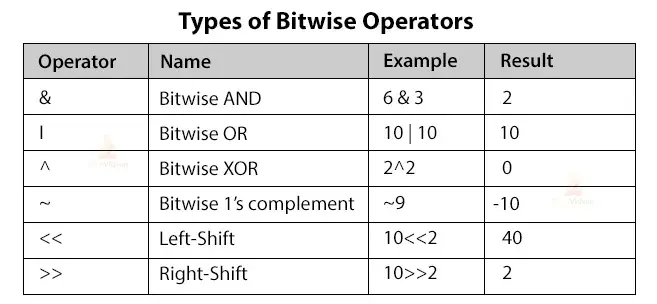
Comments